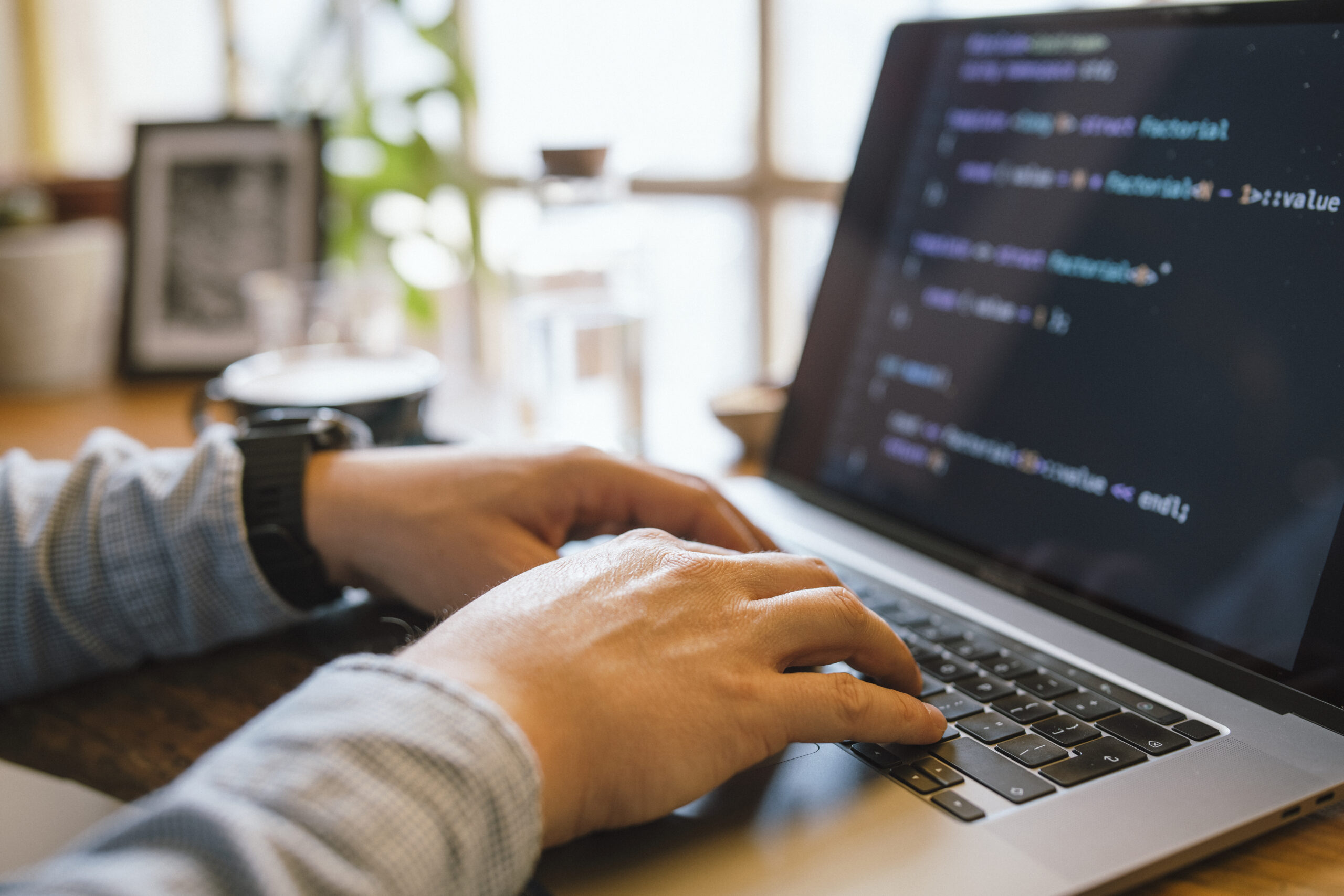
Debugging is The most vital — nonetheless often disregarded — capabilities in a very developer’s toolkit. It isn't really pretty much correcting damaged code; it’s about understanding how and why issues go Incorrect, and Understanding to Assume methodically to unravel challenges efficiently. Regardless of whether you're a newbie or even a seasoned developer, sharpening your debugging expertise can conserve several hours of annoyance and considerably transform your productiveness. Allow me to share numerous tactics that can help builders degree up their debugging sport by me, Gustavo Woltmann.
Master Your Applications
Among the fastest strategies builders can elevate their debugging expertise is by mastering the equipment they use each day. Although creating code is one Element of progress, being aware of the best way to interact with it effectively all through execution is Similarly essential. Modern progress environments arrive Outfitted with powerful debugging abilities — but numerous builders only scratch the surface area of what these tools can perform.
Just take, for instance, an Built-in Advancement Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources enable you to set breakpoints, inspect the worth of variables at runtime, stage as a result of code line by line, and in some cases modify code around the fly. When utilized effectively, they Allow you to notice precisely how your code behaves all through execution, which can be invaluable for monitoring down elusive bugs.
Browser developer applications, for example Chrome DevTools, are indispensable for entrance-stop builders. They permit you to inspect the DOM, monitor network requests, perspective true-time overall performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and community tabs can change discouraging UI problems into manageable responsibilities.
For backend or system-degree developers, resources like GDB (GNU Debugger), Valgrind, or LLDB present deep Manage in excess of functioning processes and memory management. Learning these equipment could possibly have a steeper learning curve but pays off when debugging efficiency troubles, memory leaks, or segmentation faults.
Further than your IDE or debugger, grow to be at ease with Variation control programs like Git to be aware of code record, find the precise minute bugs were being released, and isolate problematic changes.
Ultimately, mastering your equipment signifies likely beyond default settings and shortcuts — it’s about developing an intimate understanding of your advancement natural environment to make sure that when issues arise, you’re not lost in the dark. The better you know your equipment, the more time you'll be able to commit resolving the particular dilemma as an alternative to fumbling as a result of the procedure.
Reproduce the situation
One of the more important — and sometimes disregarded — measures in productive debugging is reproducing the challenge. Ahead of jumping into the code or earning guesses, builders want to make a steady atmosphere or scenario wherever the bug reliably appears. With out reproducibility, correcting a bug becomes a activity of probability, usually leading to squandered time and fragile code variations.
Step one in reproducing a challenge is gathering just as much context as you can. Inquire thoughts like: What steps led to the issue? Which natural environment was it in — advancement, staging, or production? Are there any logs, screenshots, or mistake messages? The more depth you've, the simpler it results in being to isolate the exact conditions beneath which the bug occurs.
As soon as you’ve collected enough data, attempt to recreate the situation in your local ecosystem. This could signify inputting the identical information, simulating very similar user interactions, or mimicking technique states. If The difficulty appears intermittently, look at creating automatic tests that replicate the sting conditions or state transitions included. These checks not just help expose the trouble but will also stop regressions Sooner or later.
In some cases, the issue can be environment-unique — it might take place only on selected operating programs, browsers, or less than specific configurations. Using equipment like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms may be instrumental in replicating these kinds of bugs.
Reproducing the situation isn’t simply a phase — it’s a way of thinking. It necessitates patience, observation, along with a methodical strategy. But as soon as you can continually recreate the bug, you are previously midway to repairing it. By using a reproducible circumstance, You may use your debugging applications more efficiently, examination likely fixes properly, and converse additional Plainly with the team or users. It turns an summary criticism right into a concrete problem — and that’s in which developers thrive.
Go through and Realize the Error Messages
Error messages will often be the most beneficial clues a developer has when a little something goes Completely wrong. Instead of seeing them as disheartening interruptions, builders need to find out to treat mistake messages as immediate communications from the procedure. They generally inform you what exactly occurred, where it transpired, and often even why it transpired — if you understand how to interpret them.
Begin by reading the information very carefully and in whole. Several developers, especially when less than time strain, glance at the main line and quickly begin earning assumptions. But further inside the mistake stack or logs could lie the true root bring about. Don’t just copy and paste mistake messages into engines like google — go through and understand them initially.
Split the mistake down into components. Can it be a syntax error, a runtime exception, or maybe a logic mistake? Will it level to a selected file and line amount? What module or functionality induced it? These thoughts can guidebook your investigation and issue you towards the responsible code.
It’s also valuable to understand the terminology on the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java generally follow predictable styles, and Studying to acknowledge these can drastically quicken your debugging course of action.
Some errors are obscure or generic, As well as in These scenarios, it’s crucial to examine the context where the mistake occurred. Examine linked log entries, enter values, and recent improvements in the codebase.
Don’t neglect compiler or linter warnings both. These usually precede more substantial challenges and provide hints about likely bugs.
Finally, mistake messages aren't your enemies—they’re your guides. Understanding to interpret them accurately turns chaos into clarity, serving to you pinpoint challenges quicker, minimize debugging time, and turn into a additional economical and confident developer.
Use Logging Wisely
Logging is Probably the most effective equipment in the developer’s debugging toolkit. When made use of effectively, it provides genuine-time insights into how an application behaves, assisting you comprehend what’s taking place under the hood without needing to pause execution or phase throughout the code line by line.
A superb logging approach begins with realizing what to log and at what degree. Typical logging ranges consist of DEBUG, Data, WARN, ERROR, and Lethal. Use DEBUG for specific diagnostic facts through growth, Data for common functions (like productive begin-ups), WARN for opportunity difficulties that don’t split the application, Mistake for genuine challenges, and Deadly once the method can’t continue.
Prevent flooding your logs with abnormal or irrelevant information. Too much logging can obscure vital messages and decelerate your technique. Concentrate on key gatherings, condition changes, enter/output values, and critical conclusion details with your code.
Format your log messages Plainly and regularly. Involve context, for example timestamps, request IDs, and performance names, so it’s easier to trace troubles in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs let you observe how variables evolve, what circumstances are fulfilled, and what branches of logic are executed—all with out halting This system. They’re Specially valuable in generation environments where stepping by way of code isn’t possible.
Moreover, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with checking dashboards.
Eventually, intelligent logging is about stability and clarity. That has a well-imagined-out logging tactic, you are able to decrease the time it's going to take to spot difficulties, gain deeper visibility into your apps, and Increase the overall maintainability and dependability of your respective code.
Imagine Like a Detective
Debugging is not simply a technological job—it's a kind of investigation. To correctly identify and resolve bugs, developers have to solution the process just like a detective resolving a secret. This mindset assists break down sophisticated troubles into workable sections and stick to clues logically to uncover the basis lead to.
Start out by accumulating proof. Think about the indications of the problem: mistake messages, incorrect output, or performance troubles. The same as a detective surveys a criminal offense scene, accumulate just as much appropriate data as it is possible to devoid of leaping to conclusions. Use logs, examination conditions, and person stories to piece jointly a transparent image of what’s taking place.
Subsequent, type hypotheses. Ask yourself: What could be leading to this behavior? Have any modifications lately been made into the codebase? Has this difficulty transpired just before below similar situations? The goal should be to slim down prospects and determine potential culprits.
Then, exam your theories systematically. Try and recreate the trouble in a managed ecosystem. In case you suspect a particular functionality or part, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, ask your code issues and Allow the outcomes guide you closer to the reality.
Shell out close awareness to tiny details. Bugs generally conceal during the the very least anticipated places—just like a lacking semicolon, an off-by-one particular error, or maybe a race situation. Be complete and individual, resisting the urge to patch The difficulty without thoroughly comprehending it. Temporary fixes may possibly hide the true trouble, only for it to resurface afterwards.
Lastly, hold notes on Whatever you tried and realized. Equally as detectives log their investigations, documenting your debugging approach can save time for foreseeable future challenges and aid Some others comprehend your reasoning.
By wondering similar to a detective, developers can sharpen their analytical expertise, tactic problems methodically, and turn into more practical at uncovering hidden concerns in advanced systems.
Compose Checks
Creating assessments is among the simplest ways to boost your debugging techniques and overall growth performance. Checks don't just support capture bugs early and also function a security Web that offers you assurance when making modifications in your codebase. A properly-examined software is much easier to debug mainly because it allows you to pinpoint exactly where and when an issue occurs.
Start with device checks, which deal with unique functions or modules. These small, isolated tests can quickly expose irrespective of whether a selected bit of logic is Doing work as predicted. Each time a check fails, you right away know wherever to glance, appreciably cutting down time invested debugging. Unit checks are click here In particular valuable for catching regression bugs—concerns that reappear following previously staying preset.
Upcoming, integrate integration tests and close-to-conclusion exams into your workflow. These assist make sure several areas of your application do the job alongside one another efficiently. They’re especially practical for catching bugs that come about in sophisticated systems with many elements or products and services interacting. If anything breaks, your tests can show you which Portion of the pipeline failed and less than what situations.
Crafting exams also forces you to definitely Consider critically about your code. To test a feature appropriately, you'll need to be aware of its inputs, expected outputs, and edge cases. This degree of being familiar with By natural means potential customers to higher code framework and fewer bugs.
When debugging a problem, crafting a failing check that reproduces the bug is often a powerful initial step. As soon as the examination fails consistently, you'll be able to center on fixing the bug and observe your take a look at pass when the issue is solved. This solution ensures that the identical bug doesn’t return Down the road.
In a nutshell, producing checks turns debugging from a aggravating guessing video game right into a structured and predictable process—aiding you capture additional bugs, faster and much more reliably.
Just take Breaks
When debugging a difficult problem, it’s straightforward to become immersed in the situation—gazing your monitor for hours, attempting Remedy soon after Resolution. But One of the more underrated debugging applications is solely stepping absent. Having breaks aids you reset your brain, lessen annoyance, and infrequently see The problem from the new point of view.
When you are way too near to the code for also prolonged, cognitive tiredness sets in. You could possibly get started overlooking noticeable faults or misreading code that you choose to wrote just several hours previously. In this particular condition, your brain gets to be less efficient at problem-resolving. A brief stroll, a espresso split, or perhaps switching to a different task for ten–quarter-hour can refresh your target. Numerous developers report getting the basis of a difficulty after they've taken the perfect time to disconnect, permitting their subconscious operate from the track record.
Breaks also assist prevent burnout, In particular for the duration of for a longer time debugging sessions. Sitting down in front of a monitor, mentally caught, is not just unproductive but also draining. Stepping absent enables you to return with renewed Strength along with a clearer mindset. You may perhaps out of the blue detect a lacking semicolon, a logic flaw, or maybe a misplaced variable that eluded you ahead of.
In case you’re stuck, a fantastic rule of thumb should be to set a timer—debug actively for forty five–60 minutes, then take a five–10 moment split. Use that time to maneuver about, extend, or do one thing unrelated to code. It may well come to feel counterintuitive, especially underneath restricted deadlines, but it really essentially brings about more rapidly and more effective debugging Eventually.
In short, using breaks is not really a sign of weak spot—it’s a smart system. It provides your Mind House to breathe, improves your point of view, and helps you avoid the tunnel eyesight that often blocks your progress. Debugging is actually a psychological puzzle, and relaxation is part of fixing it.
Study From Each Bug
Each and every bug you come upon is more than just A short lived setback—it's a chance to improve being a developer. Whether it’s a syntax error, a logic flaw, or possibly a deep architectural challenge, every one can educate you a thing important in the event you take some time to mirror and assess what went Completely wrong.
Start by asking your self several key concerns after the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught previously with superior techniques like device screening, code opinions, or logging? The solutions generally expose blind spots with your workflow or comprehension and make it easier to Make much better coding patterns going ahead.
Documenting bugs can even be an outstanding practice. Hold a developer journal or keep a log where you Be aware down bugs you’ve encountered, how you solved them, and what you acquired. Eventually, you’ll begin to see designs—recurring problems or typical mistakes—that you could proactively avoid.
In workforce environments, sharing Anything you've uncovered from a bug together with your friends might be Specifically potent. Whether it’s via a Slack concept, a short produce-up, or a quick knowledge-sharing session, encouraging Many others stay away from the very same problem boosts workforce effectiveness and cultivates a stronger Discovering tradition.
More importantly, viewing bugs as classes shifts your attitude from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical areas of your development journey. In spite of everything, a number of the most effective developers are usually not those who create great code, but those that consistently find out from their issues.
Ultimately, Just about every bug you repair provides a new layer to the talent set. So following time you squash a bug, have a moment to mirror—you’ll occur away a smarter, far more able developer on account of it.
Summary
Enhancing your debugging capabilities takes time, apply, and endurance — but the payoff is huge. It can make you a far more efficient, assured, and able developer. Another time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to be much better at Whatever you do.